Contents
- Matplotlib Tutorial - 파이썬으로 데이터 시각화하기
- Matplotlib 설치하기
- Matplotlib 기본 사용
- Matplotlib 숫자 입력하기
- Matplotlib 축 레이블 설정하기
- Matplotlib 범례 표시하기
- Matplotlib 축 범위 지정하기
- Matplotlib 선 종류 지정하기
- Matplotlib 마커 지정하기
- Matplotlib 색상 지정하기
- Matplotlib 그래프 영역 채우기
- Matplotlib 축 스케일 지정하기
- Matplotlib 여러 곡선 그리기
- Matplotlib 그리드 설정하기
- Matplotlib 눈금 표시하기
- Matplotlib 타이틀 설정하기
- Matplotlib 수평선/수직선 표시하기
- Matplotlib 막대 그래프 그리기
- Matplotlib 수평 막대 그래프 그리기
- Matplotlib 산점도 그리기
- Matplotlib 3차원 산점도 그리기
- Matplotlib 히스토그램 그리기
- Matplotlib 에러바 표시하기
- Matplotlib 파이 차트 그리기
- Matplotlib 히트맵 그리기
- Matplotlib 여러 개의 그래프 그리기
- Matplotlib 컬러맵 설정하기
- Matplotlib 텍스트 삽입하기
- Matplotlib 수학적 표현 사용하기
- Matplotlib 그래프 스타일 설정하기
- Matplotlib 이미지 저장하기
- Matplotlib 객체 지향 인터페이스 1
- Matplotlib 객체 지향 인터페이스 2
- Matplotlib 축 위치 조절하기
- Matplotlib 이중 Y축 표시하기
- Matplotlib 두 종류의 그래프 그리기
- Matplotlib 박스 플롯 그리기
- Matplotlib 바이올린 플롯 그리기
- Matplotlib 다양한 도형 삽입하기
- Matplotlib 다양한 패턴 채우기
- Matplotlib 애니메이션 사용하기 1
- Matplotlib 애니메이션 사용하기 2
- Matplotlib 3차원 Surface 표현하기
- Matplotlib 트리맵 그리기 (Squarify)
- Matplotlib Inset 그래프 삽입하기
Tutorials
- Python Tutorial
- NumPy Tutorial
- Matplotlib Tutorial
- PyQt5 Tutorial
- BeautifulSoup Tutorial
- xlrd/xlwt Tutorial
- Pillow Tutorial
- Googletrans Tutorial
- PyWin32 Tutorial
- PyAutoGUI Tutorial
- Pyperclip Tutorial
- TensorFlow Tutorial
- Tips and Examples
Matplotlib 다양한 패턴 채우기¶
Matplotlib 그래프 영역 또는 도형에 다양한 패턴을 채우는 방법을 소개합니다.
Keyword: 패턴 채우기, hatch, set_hatch()
■ Table of Contents
1) 기본 사용¶
예제1¶
import matplotlib.pyplot as plt
plt.style.use('default')
plt.rcParams['figure.figsize'] = (6, 5)
plt.rcParams['font.size'] = 12
x = [1, 2, 3]
y = [1, 2, 3]
fig, ((ax1, ax2), (ax3, ax4)) = plt.subplots(2, 2)
ax1.bar(x, y, color='aquamarine', edgecolor='black', hatch='/')
ax2.bar(x, y, color='salmon', edgecolor='black', hatch='\\')
ax3.bar(x, y, color='navajowhite', edgecolor='black', hatch='+')
ax4.bar(x, y, color='lightskyblue', edgecolor='black', hatch='*')
plt.tight_layout()
plt.show()
bar() 함수의 hatch는 막대 그래프의 막대 내부에 표시할 패턴을 지정합니다.
예제에서는 네가지의 서로 다른 패턴을 지정했습니다.
아래의 hatch 문자열 중에서 선택해서 사용할 수 있습니다.
{'/', '\\', '|', '-', '+', 'x', 'o', 'O', '.', '*'}
결과는 아래와 같습니다.
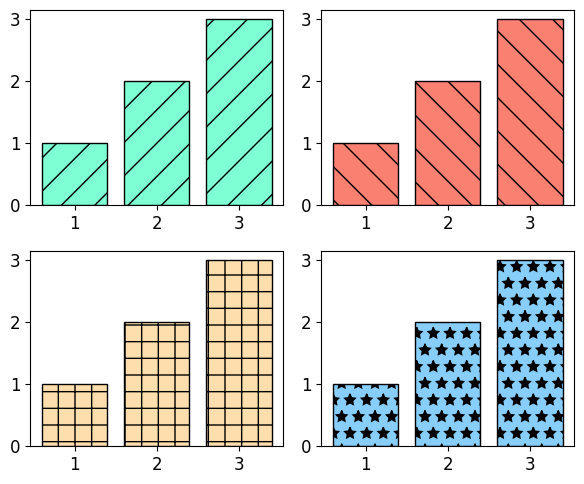
Matplotlib 다양한 패턴 채우기 - 기본 사용¶
예제2¶
import matplotlib.pyplot as plt
plt.style.use('default')
plt.rcParams['figure.figsize'] = (4, 3)
plt.rcParams['font.size'] = 12
x = [1, 2, 3]
y = [1, 2, 3]
fig, ax = plt.subplots()
bars = ax.bar(x, y, color='lightgray', edgecolor='black')
bars[0].set_hatch('x')
bars[1].set_hatch('O')
bars[2].set_hatch('.')
plt.tight_layout()
plt.show()
bar() 함수는 막대 그래프의 모든 막대 도형 (BarContainer 클래스)을 반환합니다.
set_hatch() 메서드는 각 도형에 채워질 패턴을 지정합니다.
결과는 아래와 같습니다.
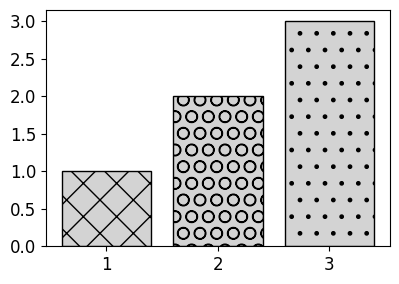
Matplotlib 다양한 패턴 채우기 - 기본 사용 2¶
2) 패턴의 밀도 지정하기¶
예제¶
import matplotlib.pyplot as plt
import numpy as np
plt.style.use('default')
plt.rcParams['figure.figsize'] = (8, 5)
plt.rcParams['font.size'] = 12
x = [1,2,3]
y = [1,2,3]
for i in range(6):
hatch_str = "/" * i
ax = plt.subplot(2, 3, i + 1)
ax.set_title("Hatch String: " + hatch_str)
bars = ax.bar(x,y,facecolor='skyblue', edgecolor='black')
for bar in bars:
bar.set_hatch(hatch_str)
plt.tight_layout()
plt.show()
hatch 문자열의 개수를 한 개 이상 입력함으로써 패턴의 밀도를 지정할 수 있습니다.
예제에서는 hatch 문자열 ‘/’에 대해 개수를 0~5개로 조절했습니다.
결과는 아래와 같습니다.
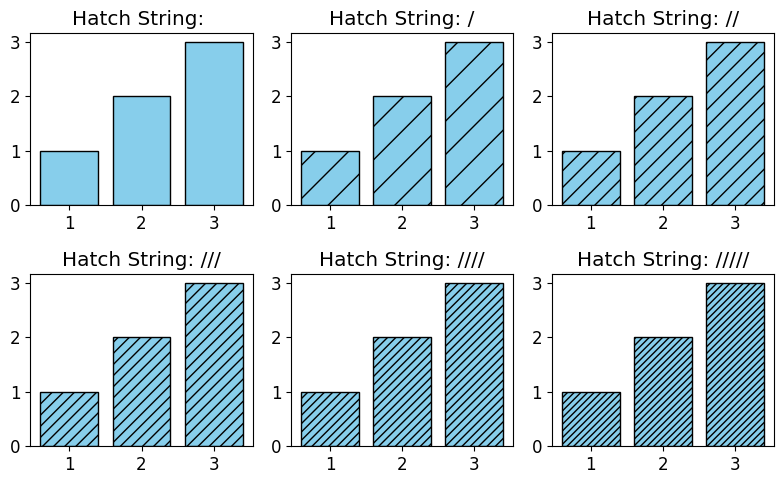
Matplotlib 다양한 패턴 채우기 - 패턴의 밀도 지정하기¶
3) 패턴의 두께 지정하기¶
예제¶
import matplotlib.pyplot as plt
import numpy as np
plt.style.use('default')
plt.rcParams['figure.figsize'] = (8, 5)
plt.rcParams['font.size'] = 12
plt.rcParams['hatch.linewidth'] = 3
x = [1,2,3]
y = [1,2,3]
for i in range(6):
hatch_str = "/" * i
ax = plt.subplot(2, 3, i + 1)
ax.set_title("Hatch String: " + hatch_str)
bars = ax.bar(x,y,facecolor='skyblue', edgecolor='black')
for bar in bars:
bar.set_hatch(hatch_str)
plt.tight_layout()
plt.show()
‘hatch.linewidth’ 속성을 사용해서 패턴의 두께를 변경할 수 있습니다.
패턴에 사용되는 선의 두께의 디폴트는 1이며, 예제에서는 3으로 변경했습니다.
결과는 아래와 같습니다.
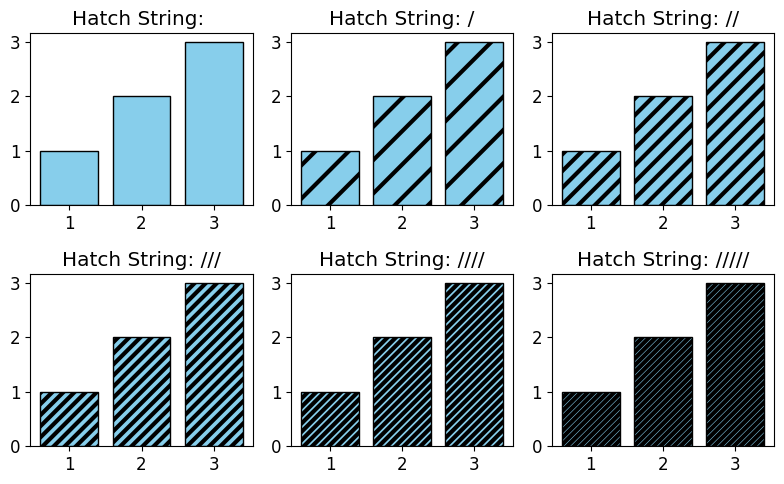
Matplotlib 다양한 패턴 채우기 - 패턴의 두께 지정하기¶
이전글/다음글
이전글 : Matplotlib 다양한 도형 삽입하기
다음글 : Matplotlib 애니메이션 사용하기 1